C++实现高性能内存池
实现一个内存池,实现默认分配器std::allocator的allocate(), dealocate(), construct(), destroy(),并且在频繁申请与释放内存的场景中拥有更优秀的性能;实现一个栈结构,其元素入栈调用分配器的allocate()申请内存,调用construct()构造对象,调用destroy()销毁对象,调用deallocate()释放内存,借助频繁的入栈出栈测试内存池性能;
内存池主要思想:
预先申请足够多的内存备用可以减少频繁申请内存的系统调用,进而提高性能;
具体实现:
- 每次内存池空间不足时(包括开始时)申请一定大小内存空间姑且称为一个Block,将每个Block划分为待存储对象大小的若干单元,每个单元称为一个Slot;
- 当一个Block全部用完时申请新的Block,且将这些Block组织成一个链表便于管理,对刚申请的Block标记其中最后一个Slot和当前已使用的Slot,当后者到达前者的位置时表明该Block已经用尽,即可以申请新的Block了;
- 对于已销毁的对象,将它们在内存池中对应的Slot组织成一个链表可称为空闲栈,表示再次可用的内存;
- 为充分利用内存,每次向内存池申请空间时先检查空闲栈是否为空,若不为空则从栈中给出一个Slot用于分配,否则检查该Block中Slot是否全部用完,若未用完则取当前Slot后一位置的Slot分配出去,否则申请新的Block;
如下:
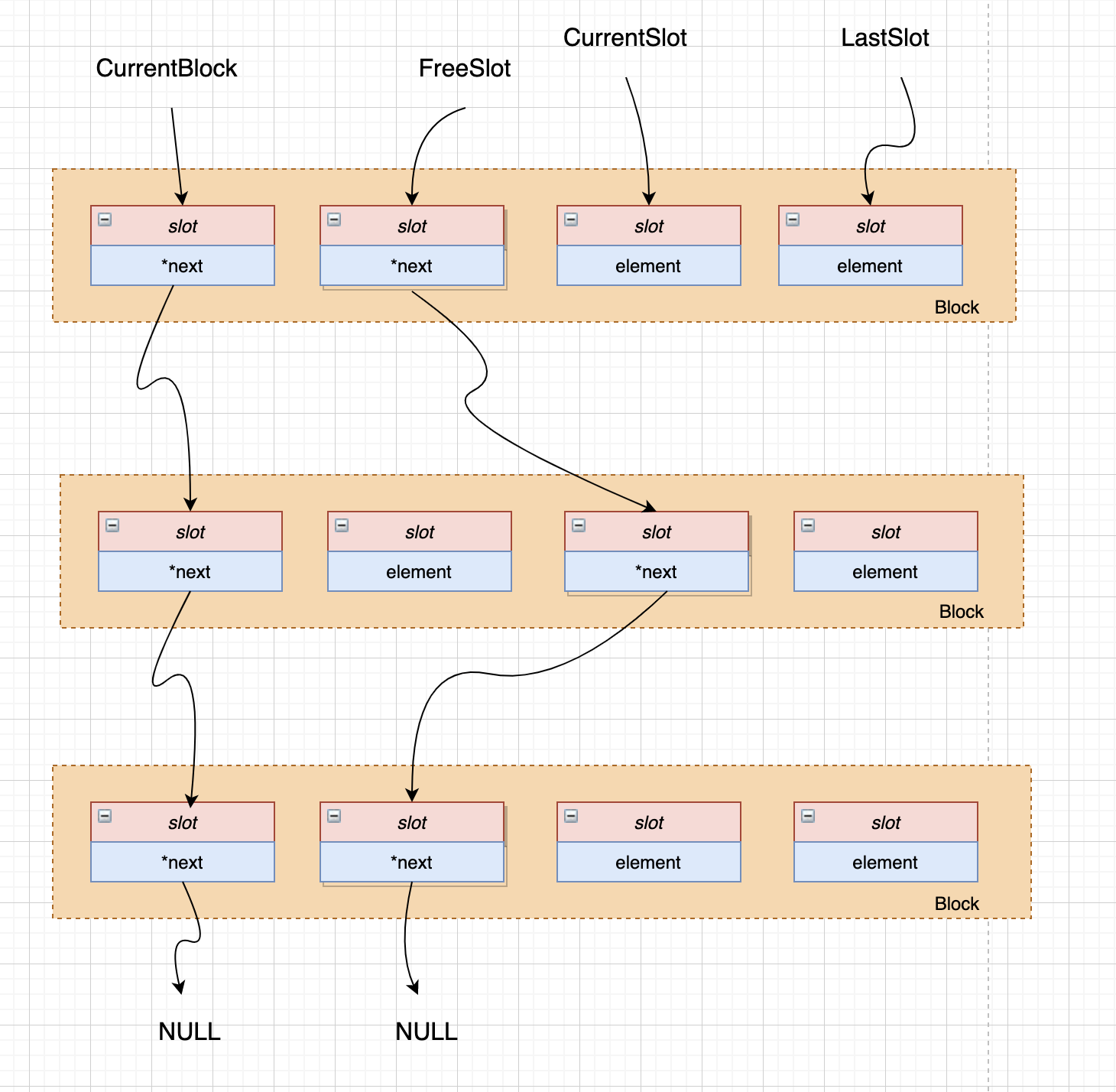
Code
整体设计:
#ifndef MEMORY_POOL_HPP
#define MEMORY_POOL_HPP
#include <climits>
#include <cstddef>
template <typename T, size_t BlockSize = 4096>
class MemoryPool
{
public:
// 使用 typedef 简化类型书写
typedef T* pointer;
// 定义 rebind<U>::other 接口
template <typename U> struct rebind {
typedef MemoryPool<U> other;
};
// 默认构造, 初始化所有的槽指针
// C++11 使用了 noexcept 来显式的声明此函数不会抛出异常
MemoryPool() noexcept {
currentBlock_ = nullptr;
currentSlot_ = nullptr;
lastSlot_ = nullptr;
freeSlots_ = nullptr;
}
// 销毁一个现有的内存池
~MemoryPool() noexcept;
// 同一时间只能分配一个对象, n 和 hint 会被忽略
pointer allocate(size_t n = 1, const T* hint = 0);
// 销毁指针 p 指向的内存区块
void deallocate(pointer p, size_t n = 1);
// 调用构造函数
template <typename U, typename... Args>
void construct(U* p, Args&&... args);
// 销毁内存池中的对象, 即调用对象的析构函数
template <typename U>
void destroy(U* p) {
p->~U();
}
private:
// 用于存储内存池中的对象槽,
// 要么被实例化为一个存放对象的槽,
// 要么被实例化为一个指向存放对象槽的槽指针
union Slot_ {
T element;
Slot_* next;
};
// 数据指针
typedef char* data_pointer_;
// 对象槽
typedef Slot_ slot_type_;
// 对象槽指针
typedef Slot_* slot_pointer_;
// 指向当前内存区块
slot_pointer_ currentBlock_;
// 指向当前内存区块的一个对象槽
slot_pointer_ currentSlot_;
// 指向当前内存区块的最后一个对象槽
slot_pointer_ lastSlot_;
// 指向当前内存区块中的空闲对象槽
slot_pointer_ freeSlots_;
// 检查定义的内存池大小是否过小
static_assert(BlockSize >= 2 * sizeof(slot_type_), "BlockSize too small.");
};
#endif // MEMORY_POOL_HPP
实现
allocate:
slot定义为联合体,具体用于存放数据还是存放指针需要转换;向操作系统申请内存直接使用operator new:
// 同一时间只能分配一个对象, n 和 hint 会被忽略
pointer allocate(size_t n = 1, const T* hint = 0) {
// 如果有空闲的对象槽,那么直接将空闲区域交付出去
if (freeSlots_ != nullptr) {
pointer result = reinterpret_cast<pointer>(freeSlots_);
freeSlots_ = freeSlots_->next;
return result;
} else {
// 如果对象槽不够用了,则分配一个新的内存区块
if (currentSlot_ >= lastSlot_) {
// 分配一个新的内存区块,并指向前一个内存区块
data_pointer_ newBlock = reinterpret_cast<data_pointer_>(operator new(BlockSize));
reinterpret_cast<slot_pointer_>(newBlock)->next = currentBlock_;
currentBlock_ = reinterpret_cast<slot_pointer_>(newBlock);
// 填补整个区块来满足元素内存区域的对齐要求
data_pointer_ body = newBlock + sizeof(slot_pointer_);
uintptr_t result = reinterpret_cast<uintptr_t>(body);
size_t bodyPadding = (alignof(slot_type_) - result) % alignof(slot_type_);
currentSlot_ = reinterpret_cast<slot_pointer_>(body + bodyPadding);
lastSlot_ = reinterpret_cast<slot_pointer_>(newBlock + BlockSize - sizeof(slot_type_));
}
return reinterpret_cast<pointer>(currentSlot_++);
}
}
constructor:
已有空间创建对象应使用placement_new:
// 调用构造函数, 使用 std::forward 转发变参模板
template <typename U, typename... Args>
void construct(U* p, Args&&... args) {
new (p) U (std::forward<Args>(args)...);
}
deallocator:
只是回收空闲Slot,不调用delete,只有内存池对象销毁时才释放其中内存;
// 销毁指针 p 指向的内存区块
void deallocate(pointer p, size_t n = 1) {
if (p != nullptr) {
// reinterpret_cast 是强制类型转换符
// 要访问 next 必须强制将 p 转成 slot_pointer_
reinterpret_cast<slot_pointer_>(p)->next = freeSlots_;
freeSlots_ = reinterpret_cast<slot_pointer_>(p);
}
}
destroy:
// 销毁内存池中的对象, 即调用对象的析构函数
template <typename U>
void destroy(U* p) {
p->~U();
}
内存池的析构函数:
// 销毁一个现有的内存池
~MemoryPool() noexcept {
// 循环销毁内存池中分配的内存区块
slot_pointer_ curr = currentBlock_;
while (curr != nullptr) {
slot_pointer_ prev = curr->next;
operator delete(reinterpret_cast<void*>(curr));
curr = prev;
}
}
用于检测性能的链栈
// StackAlloc.hpp
#ifndef STACK_ALLOC_H
#define STACK_ALLOC_H
#include <memory>
template <typename T>
struct StackNode_
{
T data;
StackNode_* prev;
};
// T 为存储的对象类型, Alloc 为使用的分配器,
// 并默认使用 std::allocator 作为对象的分配器
template <class T, class Alloc = std::allocator<T> >
class StackAlloc
{
public:
// 使用 typedef 简化类型名
typedef StackNode_<T> Node;
typedef typename Alloc::template rebind<Node>::other allocator;
// 默认构造
StackAlloc() { head_ = 0; }
// 默认析构
~StackAlloc() { clear(); }
// 当栈中元素为空时返回 true
bool empty() {return (head_ == 0);}
// 释放栈中元素的所有内存
void clear() {
Node* curr = head_;
while (curr != 0)
{
Node* tmp = curr->prev;
allocator_.destroy(curr);
allocator_.deallocate(curr, 1);
curr = tmp;
}
head_ = 0;
}
// 入栈
void push(T element) {
// 为一个节点分配内存
Node* newNode = allocator_.allocate(1);
// 调用节点的构造函数
allocator_.construct(newNode, Node());
// 入栈操作
newNode->data = element;
newNode->prev = head_;
head_ = newNode;
}
// 出栈
T pop() {
// 出栈操作 返回出栈结果
T result = head_->data;
Node* tmp = head_->prev;
allocator_.destroy(head_);
allocator_.deallocate(head_, 1);
head_ = tmp;
return result;
}
// 返回栈顶元素
T top() { return (head_->data); }
private:
allocator allocator_;
Node* head_;
};
#endif // STACK_ALLOC_H
main:
// main.cpp
#include <iostream>
#include <cassert>
#include <ctime>
#include <vector>
// #include "MemoryPool.hpp"
#include "StackAlloc.hpp"
// 根据电脑性能调整这些值
// 插入元素个数
#define ELEMS 25000000
// 重复次数
#define REPS 50
int main()
{
clock_t start;
// 使用默认分配器
StackAlloc<int, std::allocator<int> > stackDefault;
start = clock();
for (int j = 0; j < REPS; j++) {
assert(stackDefault.empty());
for (int i = 0; i < ELEMS; i++)
stackDefault.push(i);
for (int i = 0; i < ELEMS; i++)
stackDefault.pop();
}
std::cout << "Default Allocator Time: ";
std::cout << (((double)clock() - start) / CLOCKS_PER_SEC) << "\n\n";
//使用内存池
StackAlloc<int, MemoryPool<int> > stackPool;
start = clock();
for (int j = 0; j < REPS; j++) {
assert(stackPool.empty());
for (int i = 0; i < ELEMS; i++)
stackPool.push(i);
for (int i = 0; i < ELEMS; i++)
stackPool.pop();
}
std::cout << "MemoryPool Allocator Time: ";
std::cout << (((double)clock() - start) / CLOCKS_PER_SEC) << "\n\n";
return 0;
}